回溯算法
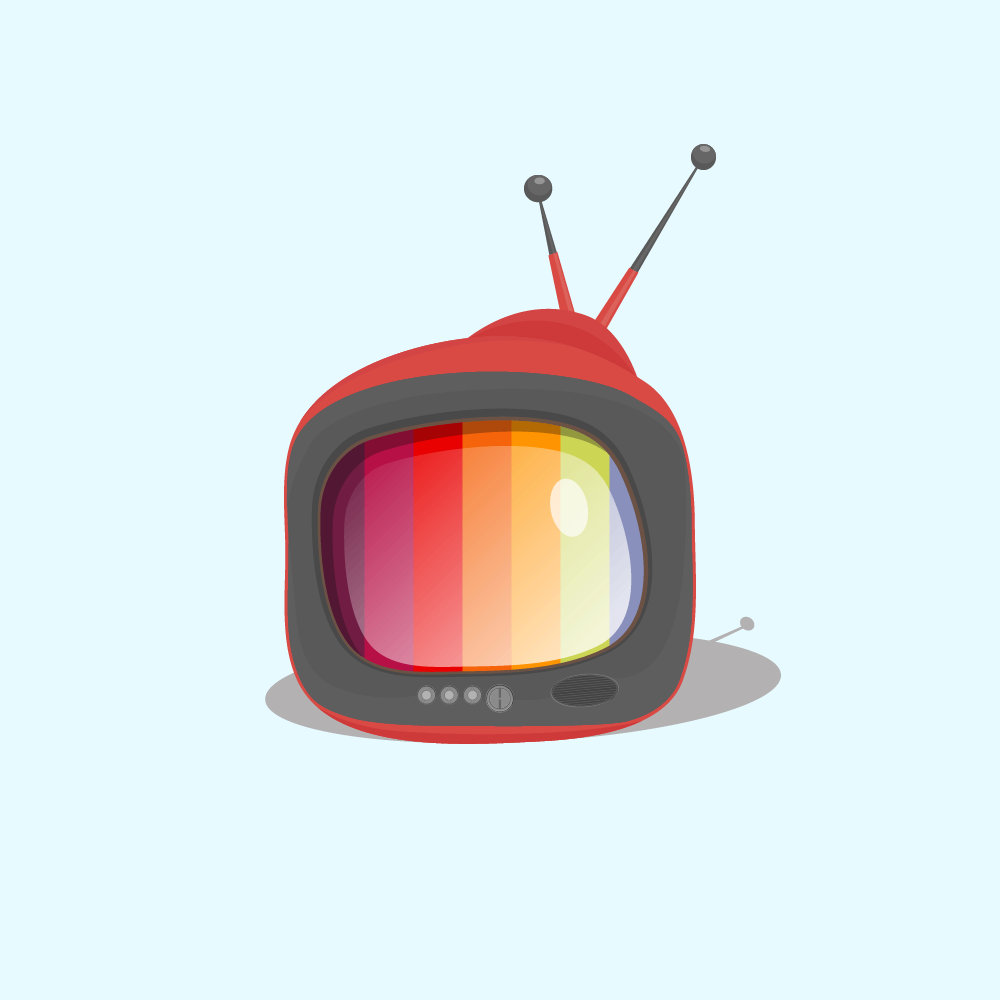
回溯算法
DreamCollector1. 简介
回溯算法(又名决策树)是一种系统地搜索问题的解的方法,也称为试探法。它的基本思想是从一条路往前走,能进则进,不能进则退回来,换一条路再试。回溯算法实际上是一个类似枚举的搜索尝试过程,主要是在搜索尝试过程中寻找问题的解。当发现已不满足求解条件时,就“回溯”返回,尝试别的路径
2. 例子
例子1:找出给定数组:[2,3,6,7]中和为:target = 7的组合,解:[[2, 2, 3], [7]]
1 | public static void backTrace(int[] nums, List<Integer> path, List<List<Integer>> result) { |
例子2:找出给定数组:[1,2,3]中不重复的排列组合,解:[[1, 2, 3], [1, 3, 2], [2, 1, 3], [2, 3, 1], [3, 1, 2], [3, 2, 1]]
1 | public static void backTrace(int[] nums, List<Integer> path, List<List<Integer>> result, int target, int start) { |
3. 总结
决策一个回溯问题,实际上就是解决一个决策树的遍历过程。需要考虑这三个问题:
- 已走路径:已做出选择,走过的路径
- 可选列表:你当前可以做的选择
- 结束条件:一般走到决策树的叶子节点,它无法再做别的条件选择
1 | //所有路径集合 |
评论
匿名评论隐私政策
✅ 你无需删除空行,直接评论以获取最佳展示效果